Google
Resident Elite
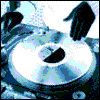 Inregistrat: acum 12 ani
Postari: 433
|
|
Simple IDA script that finds and dumps an enumeration with all the weapon ID's it can find using the mac binaries. This is my first script so I probably overcomplicated some of it, let me know if it could be improved anywhere. I only spent a few minutes browsing through all the script commands so I'm sure I missed some that could have made it smaller. Maybe I'll improve upon it later and properly sort it or something. This will work for any Source Engine mac binary but seeing as how UC doesn't have a Source Engine section, I'm just going to post it here. Anyway, here it is...
Code:
#include <idc.idc>
static FindWeaponID( FunctionAddr )
{
auto CurrentOperand;
auto FunctionEnd = FindFuncEnd( FunctionAddr );
for ( CurrentOperand = ItemHead( FunctionAddr ); CurrentOperand < FunctionEnd; CurrentOperand = ItemEnd( CurrentOperand ) )
{
if ( strstr( GetOpnd( CurrentOperand, 0 ), "eax" ) != -1 )
return GetOperandValue( CurrentOperand, 1 );
}
}
static TrimFunctionString( OriginalString )
{
auto EndPos = strstr( OriginalString, "GetWeaponID" );
auto StartPos, i;
for ( i = 0; i < strlen( OriginalString ); i ++ )
{
if ( strstr( substr( OriginalString, i, i + 1 ), "_" ) != -1 )
StartPos = i;
}
return substr( OriginalString, StartPos + 1, EndPos - 2 );
}
static main()
{
auto FunctionAddr, FuncName, StrCmp;
Message( "enum EWeaponID {\n" );
for ( FunctionAddr = NextFunction( 0 ); FunctionAddr != BADADDR; FunctionAddr = NextFunction( FunctionAddr ) )
{
FuncName = GetFunctionName( FunctionAddr );
StrCmp = strstr( FuncName, "GetWeaponID" );
if ( StrCmp != -1 )
Message( "\t%s = %i,\n", TrimFunctionString( FuncName ), FindWeaponID( FunctionAddr ) );
}
Message( "};" );
} |
Code:
// L4D2
enum EWeaponID {
WeaponCSBase = 0,
AssaultRifle = 5,
AutoShotgun = 4,
BaseBackpackItem = 0,
BoomerClaw = 41,
CarriedProp = 16,
Chainsaw = 20,
ChargerClaw = 40,
ColaBottles = 28,
FireworkCrate = 29,
FirstAidKit = 12,
GasCan = 16,
Gnome = 27,
GrenadeLauncher = 21,
HunterClaw = 39,
Adrenaline = 23,
ItemAmmoPack = 22,
ItemDefibrillator = 24,
ItemUpgradePackExplosive = 31,
ItemUpgradePackIncendiary = 30,
VomitJar = 25,
JockeyClaw = 44,
Molotov = 13,
OxygenTank = 18,
PainPills = 15,
PipeBomb = 14,
Pistol = 1,
MagnumPistol = 32,
PropaneTank = 17,
PumpShotgun = 3,
AK47 = 26,
Desert = 9,
M60 = 37,
SG552 = 34,
Chrome = 8,
SPAS = 11,
MP5 = 33,
Silenced = 7,
SmokerClaw = 42,
SniperRifle = 6,
AWP = 35,
Military = 10,
Scout = 36,
SpitterClaw = 43,
SubMachinegun = 2,
TankClaw = 38,
TerrorMeleeWeapon = 19,
WeaponSpawn = 8,
}; |
Code:
// TF2
enum EWeaponID {
TFBaseProjectile = 8,
EnergyRing = 79,
TFWeaponBase = 0,
TFWeaponBaseGrenadeProj = 0,
TFWeaponBaseMelee = 0,
TFBaseRocket = 22,
TFBat = 1,
Fish = 72,
Wood = 2,
Giftwrap = 82,
TFBonesaw = 11,
TFBottle = 3,
TFStickBomb = 74,
TFBuffItem = 62,
TFClub = 5,
TFCompoundBow = 61,
TFFireAxe = 4,
TFFists = 8,
TFFlameThrower = 25,
TFFlameRocket = 52,
TFFlareGun = 58,
Revenge = 84,
TFGrenadeLauncher = 23,
TFGrenadePipebombProjectile = 8,
TFWeaponInvis = 57,
TFJar = 60,
TFJarMilk = 70,
JarMilk = 40,
TFKnife = 7,
TFLaserPointer = 67,
TFLunchBox = 59,
TFMechanicalArm = 80,
WeaponMedigun = 50,
TFMinigun = 18,
TFParticleCannon = 79,
TFWeaponPDA = 45,
Build = 46,
Destroy = 47,
Spy = 48,
TFPipebombLauncher = 24,
TFPistol = 41,
Scout = 42,
ScoutPrimary = 71,
ScoutSecondary = 75,
TFRaygun = 78,
TFDRGPomson = 81,
TFRevolver = 43,
TFRocketLauncher = 22,
DirectHit = 65,
TFCrossbow = 73,
TFShotgun = 12,
Revenge = 69,
TFScatterGun = 16,
Soldier = 13,
HWG = 14,
Pyro = 15,
TFSodaPopper = 76,
TFShovel = 9,
TFSMG = 19,
TFSniperRifle = 17,
TFSniperRifleDecap = 77,
ZNK30CTFDecapitationMeleeWeaponBase = 64,
TFSyringeGun = 20,
TFWrench = 10,
TFWeaponBuilder = 49,
}; |
Code:
// CSS
enum EWeapnID {
AK47 = 27,
WeaponAug = 8,
WeaponAWP = 17,
WeaponCSBase = 0,
C4 = 6,
DEagle = 25,
WeaponElite = 10,
WeaponFamas = 15,
WeaponFiveSeven = 11,
Flashbang = 24,
WeaponG3SG1 = 23,
WeaponGalil = 14,
WeaponGlock = 2,
HEGrenade = 4,
Knife = 28,
WeaponM249 = 19,
WeaponM3 = 20,
WeaponM4A1 = 21,
WeaponMAC10 = 7,
WeaponMP5Navy = 18,
WeaponP228 = 1,
WeaponP90 = 29,
WeaponScout = 3,
WeaponSG550 = 13,
WeaponSG552 = 26,
SmokeGrenade = 9,
WeaponTMP = 22,
WeaponUMP45 = 12,
WeaponUSP = 16,
WeaponXM1014 = 5,
}; |
|
|